Metrics
Built-in support for keeping track of key metrics
Having easy access to key metrics is a critical part of application observability. Encore solves this by providing automatic dashboards of common application-level metrics for each service.
Encore also makes it easy to define custom metrics for your application. Once defined, custom metrics are automatically displayed on metrics page in the Cloud Dashboard.
By default, Encore also exports metrics data to your cloud provider's built-in monitoring service.
Defining custom metrics
Define custom metrics by importing the encore.dev/metrics
package and
create a new metric using one of the metrics.NewCounter
or metrics.NewGauge
functions.
For example, to count the number of orders processed:
import "encore.dev/metrics"
var OrdersProcessed = metrics.NewCounter[uint64]("orders_processed", metrics.CounterConfig{})
func process(order *Order) {
// ...
OrdersProcessed.Increment()
}
Metric types
Encore currently supports two metric types: counters and gauges.
Counters, like the name suggests, measure the count of something. A counter's value must always increase, never decrease. (Note that the value gets reset to 0 when the application restarts.) Typical use cases include counting the number of requests, the amount of data processed, and so on.
Gauges measure the current value of something. Unlike counters, a gauge's value can fluctuate up and down. Typical use cases include measuring CPU usage, the number of active instances running of a process, and so on.
For information about their respective APIs, see the API documentation for Counter and Gauge.
Defining labels
Encore's metrics package provides a type-safe way of attaching labels to metrics.
To define labels, create a struct type representing the labels and then use metrics.NewCounterGroup
or metrics.NewGaugeGroup
:
type Labels struct {
Success bool
}
var OrdersProcessed = metrics.NewCounterGroup[Labels, uint64]("orders_processed", metrics.CounterConfig{})
func process(order *Order) {
var success bool
// ... populate success with true/false ...
OrdersProcessed.With(Labels{Success: success}).Increment()
}
Take care
Each combination of label values creates a unique time series tracked in memory and stored by the monitoring system. Using numerous labels can lead to a combinatorial explosion, causing high cloud expenses and degraded performance.
As a general rule, limit the unique time series to tens or hundreds at most, rather than thousands.
Integrations with third party observability services
To make it easy to use a third party service for monitoring, we're adding direct integrations between Encore and popular observability services. This means you can send your metrics directly to these third party services instead of your cloud provider's monitoring service.
Grafana Cloud
To send metrics data to Grafana Cloud, you first need to Add a Grafana Cloud Stack to your application.
Open your application in the Encore Cloud dashboard, and click on Settings in the main navigation. Then select Grafana Cloud in the settings menu and click on Add Stack.
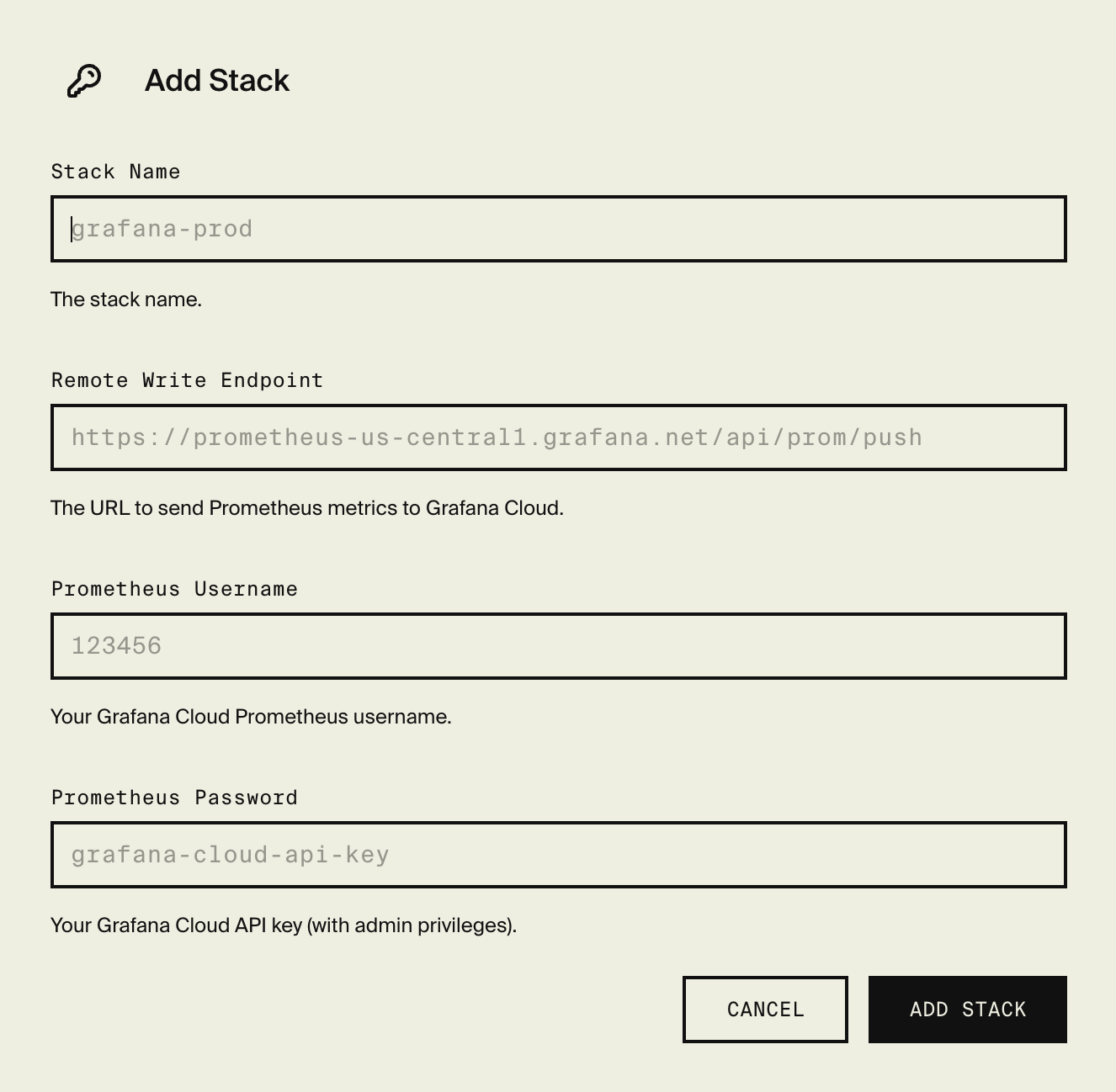
Next, open the environment Overview for the environment you wish to sent metrics from and click on Settings. Then in the Sending metrics data section, select your Grafana Cloud Stack from the drop-down and save.
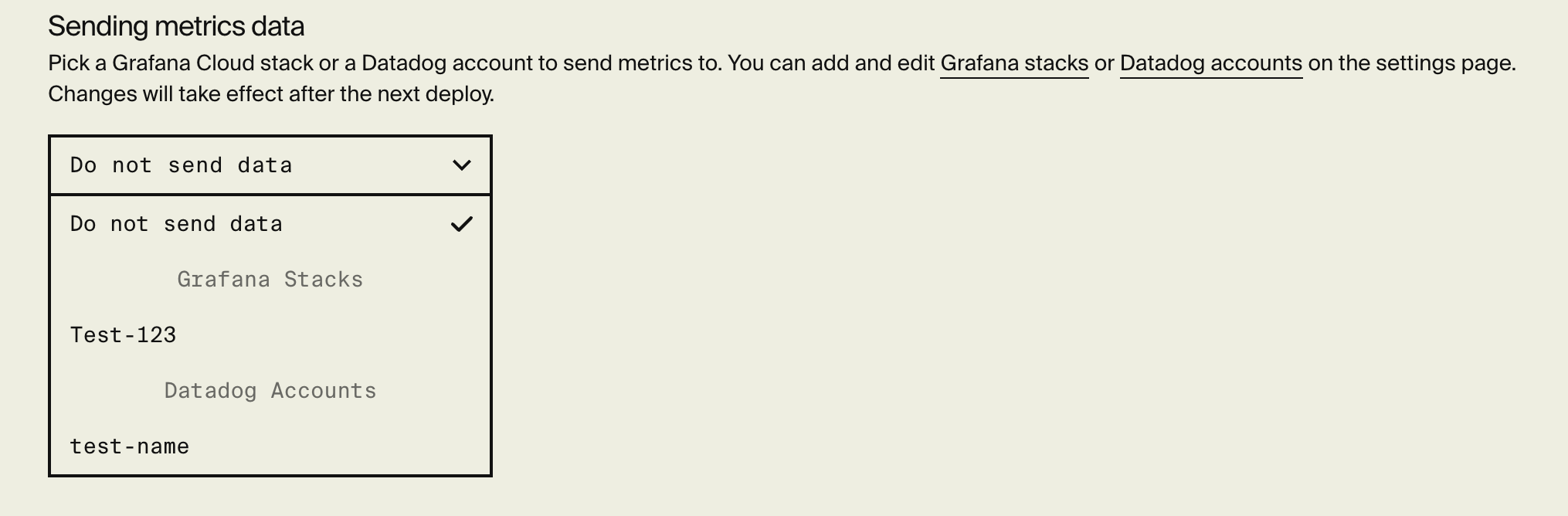
That's it! After your next deploy, Encore will start sending metrics data to your Grafana Cloud Stack.
Datadog
To send metrics data to Datadog, you first need to add a Datadog Account to your application.
Open your application in the Encore Cloud dashboard, and click on Settings in the main navigation. Then select Datadog in the settings menu and click on Add Account.
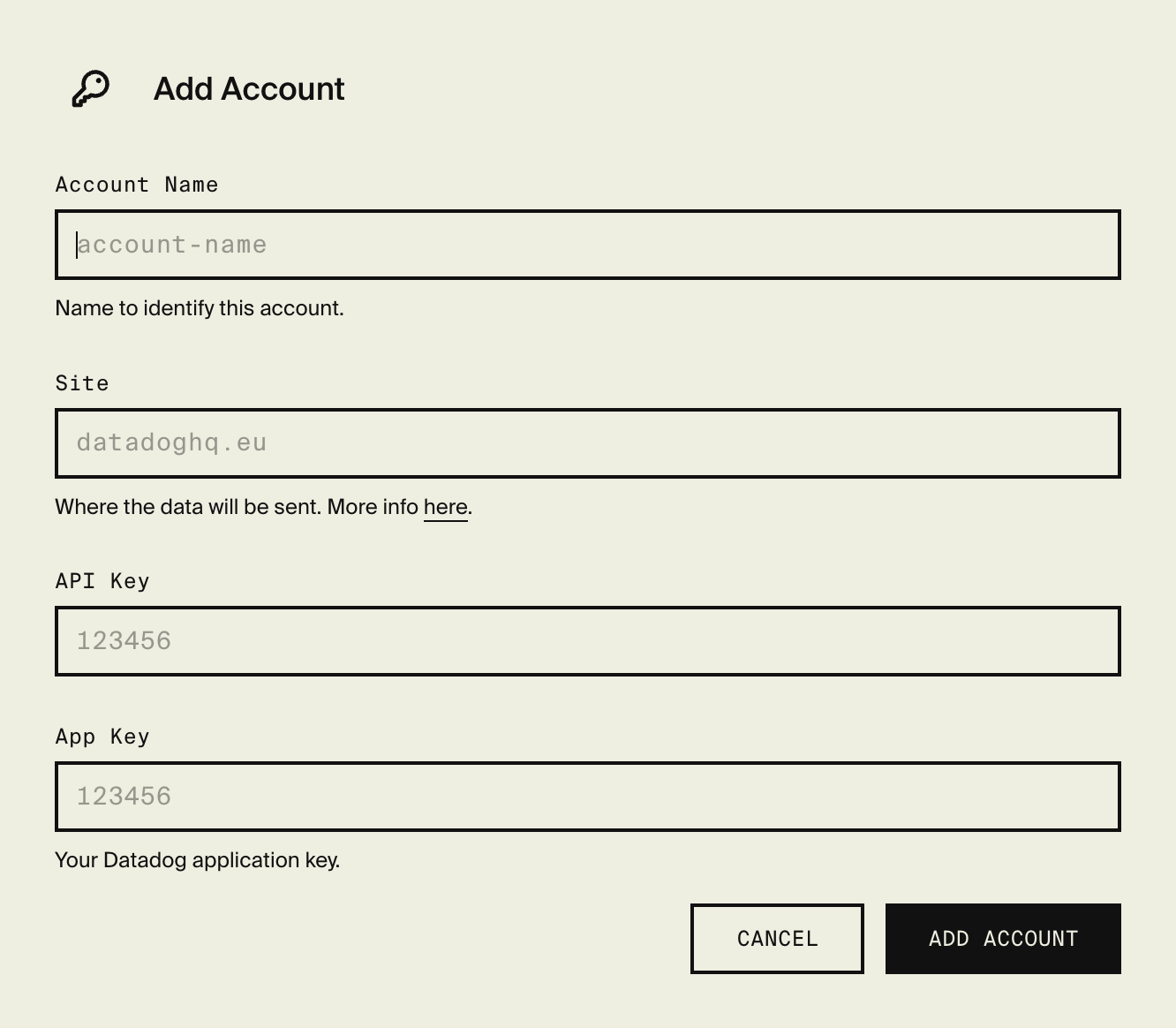
Next, open the environment Overview for the environment you wish to sent metrics from and click on Settings. Then in the Sending metrics data section, select your Datadog Account from the drop-down and save.
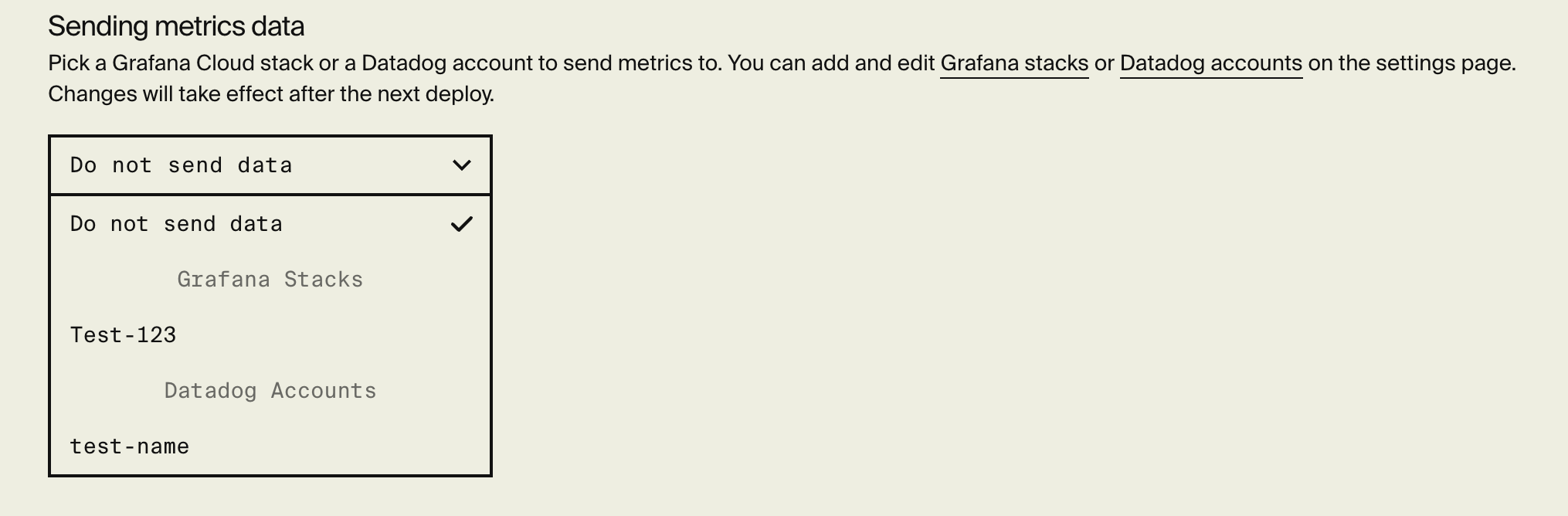
That's it! After your next deploy, Encore will start sending metrics data to your Datadog Account.